Learn JavaScript Basics in 5 Minutes
As of 2022, there is no better time to learn JavaScript.
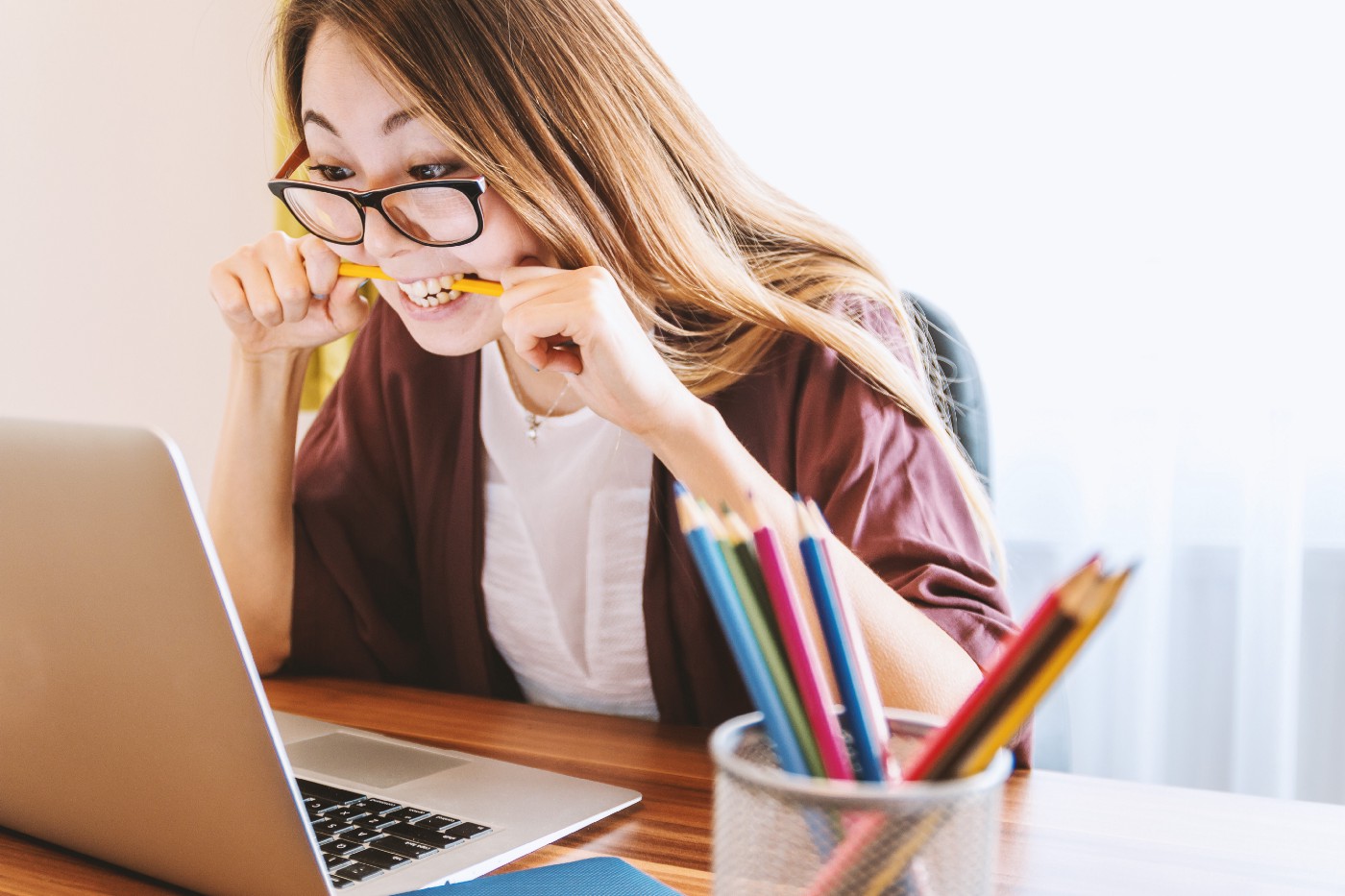
Photo by JESHOOTS.COM on Unsplash
Introduction
According to the Stack Overflow developer survey, JavaScript was the most popular programming language of 2019. It has rapidly evolved and is not even close to the language it was five years ago. Almost everything has changed for the better — from where it can run to the actual syntax of the language. As of 2020, there is no better time to learn this dynamic language and hence, today we will talk about the simplest approach to learning JavaScript.
How does JavaScript work?
When you open any website on the Internet, your computer receives a lot of files, including at least one HTML file. This HTML file may contain a [
Among other things, client-side code can:
- Change the display on the interface;
- Send data to the server;
- Fetch more data from the server.
One might have heard of client-side frameworks like — React, Angular, or Vue which are built on top of JavaScript to make understanding the client-side code a lot easier and maintainable.
Traditionally, JavaScript is an interpreted language, which means that the code is read line by line rather than having to go through a compiled step where it’s then converted to machine code.
Browsers have actually added something called the Just in Time (JIT) compiler to speed up their engines. While JavaScript was known as a slow language in the past, computers are so fast now that this statement is no longer true. JavaScript is doing pretty much everything these days, from running servers to machine learning and much more. We can do a lot of stuff on the backend now with the JavaScript environment known as Node.js. That means we can write APIs, talk to databases, and other servers, all with JavaScript. Node.js uses the same V8 engine as Chrome, and hence it’s lightning fast.
Syntax of JavaScript
JavaScript variables hold either single cell primitives or compound data.
- Primitives are usually numbers, boolean or strings of text;
- Compound data are held by arrays and objects;
In modern JavaScript, we use the keywords let and const to declare variables. In case you plan to change it later, you may use let, otherwise use const. Arrays are also known as lists in other languages, where they are just multi-celled ordered clusters of data. Objects, also known as dictionaries in other languages, are pretty much what they sound like. You can give it a word and it retrieves the definition. Examples:
const myList = ['John', 33, true];
console.log(myList[0]); // 'John'
const myDict = {name: 'John', age: 33, active: true};
console.log(myDict.name); // 'John'
The DOM (Document Object Model) is the most important object in JavaScript. It’s a JavaScript representation of the structure of any HTML, and is present in the variable document when we load the page. This document object contains many element objects. Through the DOM we can search for specific elements, add Event Listeners or change how the page looks like. All this is possible through a set of methods called the “Browser API”.
JavaScript is a C-like language, which means that it borrowed most of its syntax from C. This includes if statements, for loops, while loops, and more. When it comes to loops, most commonly we’ll be using the for in loop to iterate over an object, and the regular for loop to iterate over an array.
Alternatively, functions are just any code we want to use more than once. We declare these with the special keyword function followed by whatever name we want to give our function, along with the parameter inputs through our function.
function add(first, second) {
return first + second;
}
These can be considered as variables that are set later when we use our functions. We also want to return something which is the output or result of our function. Call a function with its name and pass in the input arguments to get the output.
console.log(add(1, 2)); // prints 3
Methods are the same as functions, except that they’re attached with different data types. We call these methods with the “dot” notation.
Examples:
'John'.toUpperCase();
(300).toFixed(2);
['ant', 'bison', 'camel'].indexOf('bison');
Functions can also be written with the parameters on the left, and this returns the result automatically in one line. These are known as arrow functions.
(a, b) => a + b
Arrow functions are generally used in array methods which take a function as an argument and run that function on every item in the array.
Another large segment of JavaScript is known as asynchronous programming. This is also known as performing “non-deterministic” operations, such as a network request to a server to fetch more data. In this case, we don’t know when the request is going to return, or if it’s going to fail. Therefore, it’s required to handle both of these cases. Async programs have evolved a lot over the years in JavaScript, but the current standard is async/await. All you have to do is add the await keyword and it will pause the program upon sending a request, until the request either comes back or fails.
await function fetchData(url) {
const response = await fetch(url);
}
At this point, we can also talk about TypeScript which compiles into normal JavaScript. Vanilla JavaScript has what’s known as dynamic types or no types and TypeScript gives static typing. This means we have to be specific about what kind of value is in a variable. This may seem like additional work but it actually helps us catch tons of errors.
Finally, there’s the npm (Node Package Manager). If you need some code, someone else has already written that particular code. Therefore, it can be downloaded as a module and you can be sure that it’s well tested and bug free.